Hi @Piriziwè ,
Here is an example code that you may use and that puts vertical arrows on every atom.
void SEArrowDrawerVisualModel::display() {
SBNodeIndexer nodes;
SAMSON::getActiveDocument()->getNodes(nodes, SBNode::IsType(Type::Atom));
unsigned int nCylinders = 2 * nodes.size();
unsigned int nPositions = 4 * nodes.size();
unsigned int* indexData = new unsigned int[2 * nCylinders];
float *positionData = new float[3 * nPositions];
float *radiusData = new float[2 * nCylinders];
unsigned int *capData = new unsigned int[2 * nCylinders];
float *colorData = new float[4 * 2 * nCylinders];
unsigned int *flagData = new unsigned int[2 * nCylinders];
int atomCounter = 0;
SB_FOR(SBNode* n, nodes) {
SBAtom* atom = (SBAtom*) n;
SBVector3 arrowDirection(1, 0, 0);
SBQuantity::length arrowLength(60);
positionData[atomCounter * 12 + 0] = (atom->getPosition().v[0] + arrowDirection.v[0] * SAMSON::getAtomRadius()).getValue();
positionData[atomCounter * 12 + 1] = (atom->getPosition().v[1] + arrowDirection.v[1] * SAMSON::getAtomRadius()).getValue();
positionData[atomCounter * 12 + 2] = (atom->getPosition().v[2] + arrowDirection.v[2] * SAMSON::getAtomRadius()).getValue();
positionData[atomCounter * 12 + 3] = (atom->getPosition().v[0] + arrowDirection.v[0] * (SAMSON::getAtomRadius() + 0.3 * arrowLength)).getValue();
positionData[atomCounter * 12 + 4] = (atom->getPosition().v[1] + arrowDirection.v[1] * (SAMSON::getAtomRadius() + 0.3 * arrowLength)).getValue();
positionData[atomCounter * 12 + 5] = (atom->getPosition().v[2] + arrowDirection.v[2] * (SAMSON::getAtomRadius() + 0.3 * arrowLength)).getValue();
radiusData[atomCounter * 4 + 0] = 0;
radiusData[atomCounter * 4 + 1] = 10;
positionData[atomCounter * 12 + 6] = (atom->getPosition().v[0] + arrowDirection.v[0] * (SAMSON::getAtomRadius() + 0.3 * arrowLength)).getValue();
positionData[atomCounter * 12 + 7] = (atom->getPosition().v[1] + arrowDirection.v[1] * (SAMSON::getAtomRadius() + 0.3 * arrowLength)).getValue();
positionData[atomCounter * 12 + 8] = (atom->getPosition().v[2] + arrowDirection.v[2] * (SAMSON::getAtomRadius() + 0.3 * arrowLength)).getValue();
positionData[atomCounter * 12 + 9] = (atom->getPosition().v[0] + arrowDirection.v[0] * (SAMSON::getAtomRadius() + arrowLength)).getValue();
positionData[atomCounter * 12 + 10] = (atom->getPosition().v[1] + arrowDirection.v[1] * (SAMSON::getAtomRadius() + arrowLength)).getValue();
positionData[atomCounter * 12 + 11] = (atom->getPosition().v[2] + arrowDirection.v[2] * (SAMSON::getAtomRadius() + arrowLength)).getValue();
radiusData[atomCounter * 4 + 2] = 2;
radiusData[atomCounter * 4 + 3] = 2;
indexData[atomCounter * 4 + 0] = 4 * atomCounter + 0;
indexData[atomCounter * 4 + 1] = 4 * atomCounter + 1;
indexData[atomCounter * 4 + 2] = 4 * atomCounter + 2;
indexData[atomCounter * 4 + 3] = 4 * atomCounter + 3;
capData[atomCounter * 4 + 0] = 1;
capData[atomCounter * 4 + 1] = 1;
capData[atomCounter * 4 + 2] = 1;
capData[atomCounter * 4 + 3] = 1;
colorData[atomCounter * 16 + 0 ] = 1;
colorData[atomCounter * 16 + 1 ] = 1;
colorData[atomCounter * 16 + 2 ] = 1;
colorData[atomCounter * 16 + 3 ] = 1;
colorData[atomCounter * 16 + 4 ] = 1;
colorData[atomCounter * 16 + 5 ] = 1;
colorData[atomCounter * 16 + 6 ] = 1;
colorData[atomCounter * 16 + 7 ] = 1;
colorData[atomCounter * 16 + 8 ] = 1;
colorData[atomCounter * 16 + 9 ] = 1;
colorData[atomCounter * 16 + 10] = 1;
colorData[atomCounter * 16 + 11] = 1;
colorData[atomCounter * 16 + 12] = 1;
colorData[atomCounter * 16 + 13] = 1;
colorData[atomCounter * 16 + 14] = 1;
colorData[atomCounter * 16 + 15] = 1;
unsigned int flag = (atom->isSelected() ? 1 : 0) + (atom->isHighlighted() ? 2 : 0);
flagData[atomCounter * 4 + 0] = flag;
flagData[atomCounter * 4 + 1] = flag;
flagData[atomCounter * 4 + 2] = flag;
flagData[atomCounter * 4 + 3] = flag;
atomCounter++;
}
SAMSON::displayCylinders(nCylinders, nPositions, indexData, positionData, radiusData, capData, colorData, flagData);
delete[] indexData;
delete[] radiusData;
delete[] capData;
delete[] colorData;
delete[] flagData;
}
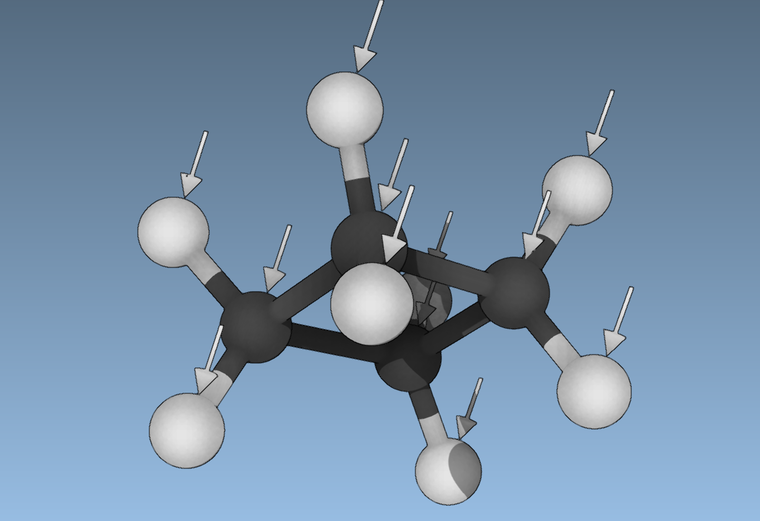